React Component Lifecycle Methods
The Component Lifecycle
The component lifecycle goes through the following phase
- Mounting
- Updating
- Unmounting
Mounting
The component rendered to the DOM for the first time. This is called mounting. These methods are called in the following order when an instance of a component is being created and inserted into the DOM.
constructor()
static getDerivedStateFromProps()
rarely case userender()
componentDidMount()
Updating
An update can be caused by changes to props or state. These methods are called in the following order when a component is being re-rendered
static getDerivedStateFromProps()
rarely case useshouldComponentUpdate()
rarely case userender()
getSnapshotBeforeUpdate()
rarely case usecomponentDidUpdate()
Unmounting
When component removed from DOM. This is called unmounting. Below method is called in this phase.
componentWillUnmount()
Component Lifecycle Diagram
React Version 16.4
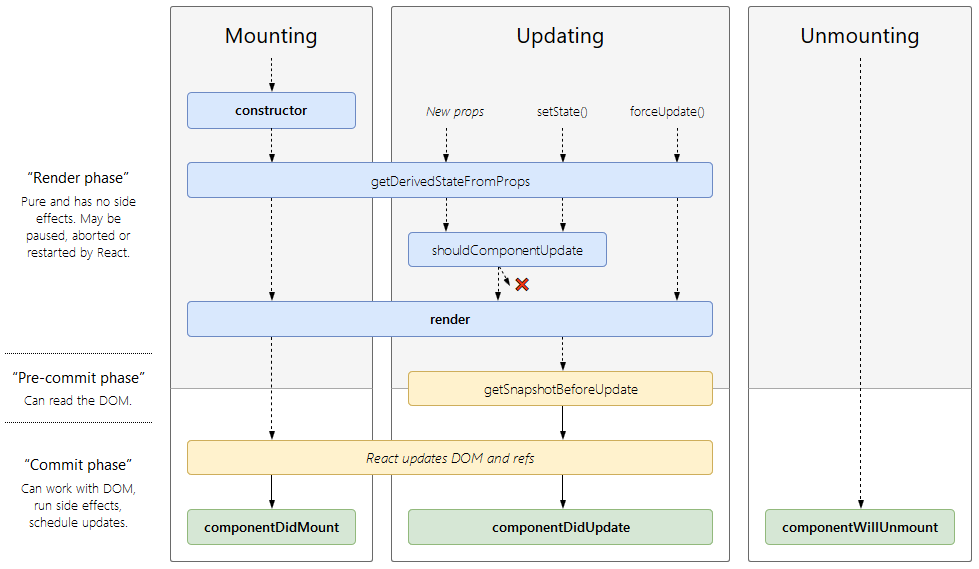
Lifecycle Methods
constructor()
The constructor for a React component is called before it is mounted. The constructor call only once in whole lifecycle. You and set initial value for this component.
Constructors are only used for two purposes 1. Initializing local state by assigning an object to this.state 2. Binding event handler methods to an instance.
static getDerivedStateFromProps()
It is invoked before calling render method. This method is used when state depends on props, whenever props is changed, then state automatically will be changed. getDerivedStateFromProps method use for both phase mounting and updating of the component. If null is returned then nothing is changed in the state.
render()
This method is the only required method in a class component. The render() function should be pure, meaning that it does not modify component state, which means it returns the same output each time it’s invoked.
componentDidMount()
This is invoked immediately after the component is mounted. If you load data using api then it right place for request data using api.
shouldComponentUpdate()
This is invoked before rendering when new props or state are being received. This methods return true or false according the component re-render or skipped. By default, this method returns true. This method is not called for the initial render.
getSnapshotBeforeUpdate()
This is invoked before the most recently rendered output is committed to the DOM. It is capture some information from the DOM before it is potentially changed. If getSnapshotBeforeUpdate method return any value the same passed as a parameter to componentDidUpdate().
componentDidUpdate()
This method immediately executed on the DOM when the component has been updated. Update occurs by changing state and props. This method is not called for the initial render. This is a good place for compare the current props to previous props.
componentWillUnmount()
This method immediately executed when the component is unmounted and destroy from the DOM. Means this method is called when a component is being removed from the DOM.
Explain Component Lifecycle with Example
Note: This is not an e-commerce shopping cart features. This example only for explaining component lifecycle flow.
Create a file src/Product.js
Create a file src/App.js and an import Product component in this App component.
Import App component into src/Index.js
Index.js has rendered in the DOM root node.
This lesson also available on YouTube
Please leave comments
5 Comments
